Pages
Nucular Secrets
LSL help: any question is fair game!
Monday, February 28, 2011
Let's Talk About Lag
Ever been on SL and wonder why everything's so slow? Well I stumbled on this website that will now show you why everything sucks. So before you start slitting your wrists, check this out!
http://www.akamai.com/html/technology/dataviz1.html
Oh, I should mention that this only shows general network stuffs, SL could be having problems of their own, like the hamsters aren't running fast enough.
Saturday, November 27, 2010
Detecting A Name
touch_start(integer total_number)
{
llOwnerSay(llDetectedName(0));
}
In this example I put it in a touch event, but it could also go in a collision event. You can also set things to be run by the owner of the object only by using the following snippet. Anything between the brackets will be owner only:
if(llDetectedKey(0) == llGetOwner()){
}
Sunday, September 19, 2010
The Sigil Stone
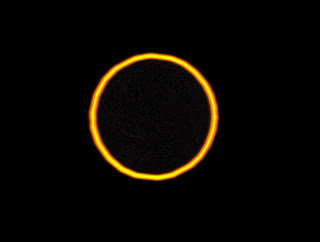
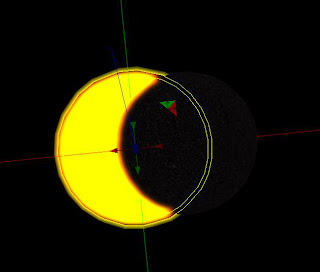
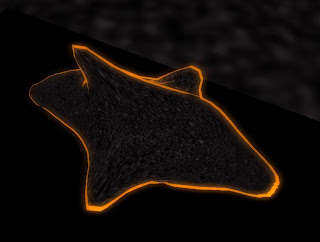
I have been asked a few times about how this is done so I thought I'd do a blog post (I need more stuff to blog about anyway, merp). I'm sure you've probably seen this kind of effect before, it's mostly used with cartoon avatars, to give them that black drawn outline look. Here's how it's done.
First take the prim you want to use for the inside, then copy it and make it slightly larger than the inside prim. Make it as hollow as it can get, then texture the outside invisible, so you can see the inside, and retexture the inside of the hollow prim.
You can do this with sculptie prims too but it's done slightly differently since you can't make them hollow. Create the two prims in the same way, so that one is slightly larger than the other. This time, on the larger prim, go to the "object" tab in the build menu, and under the sculptie texture input, select "inside out".
Monday, August 16, 2010
Setting up your own Open Sim
Well, a few months ago I had tried this and became deeply, deeply frustrated. It seemed nothing I did was working, and the situation was hopeless, so I stopped trying to set up my own Open Sim on my local machine. Yesterday however someone asked me if I ever got it working, and I told them no, but maybe I could look into it again.
So I did. Only to find that my first google search and attempt at setting it up again were successful. I love when things work out :)
Here are the instructions that I followed :
http://arianeb.wordpress.com/2010/04/14/setting-up-a-simple-open-sim-sandbox-on-your-hard-drive/
Saturday, August 14, 2010
Allow Inventory Drop
If it's set to true, anyone can drop inventory in, any object type except for scripts. The exception to this is that scripts can be transferred from object to object if llAllowInventoryDrop() is true, but the scripts are transferred in a non-running state, meaning they either need to be compiled again, or the object needs to be saved and re-rezzed.
Monday, August 02, 2010
Skinning Cats
Here are a few ways to do a click and drag thinger with a HUD. They all have their pros and cons.
1. Dragging out a selection box texture on a prim. This requires 2 prims, one for the actual box and one for an image under it (so you can see the box).
Pro: Looks cool and will drag a 2D selection box on the surface of a prim.
Con: Only affects the texture and doesn't move a prim. Well I guess that's not a con if you're into that kind of thing.
You can find the code for that at the LSL code receptacle on the wiki. http://wiki.secondlife.com/wiki/ClickAndDrag
2. Dragging a prim around using llDetectedTouchPos
Pros: Only uses one prim and can be dragged as far as you want in any direction.
Cons: Can only be dragged so fast because if you move your mouse off the surface of the prim, the touch event will stop and the prim won't move anymore. *Edit* Tahlie pointed out to me that the event doesn't actually stop, just that the prim isn't getting information on where to move anymore, and you can keep moving the prim if you move your mouse back over the prim.
//Written and given permission to duplicate by Tahlie Teskat3. Using a the UV coordinates of the texture of an invisible prim to drag another prim.
key owner;
// For move Function
vector LastTouchPos;
default {
// Reset Security
state_entry() {
owner = llGetOwner();
}
on_rez(integer rezzed) {
if (owner != llGetOwner()) llResetScript();
}
link_message(integer sender_num, integer num, string str, key id) {
if (num == 666 && str == "reset") llResetScript();
}
// Reset Security
touch_start(integer total) {
LastTouchPos = llDetectedTouchPos(0);
}
touch(integer total) {
vector delta;
vector touchPos = llDetectedTouchPos(0);
if (touchPos != <0.0,0.0,0.0>) delta = touchPos - LastTouchPos;
if (delta != <0.0,0.0,0.0>) llSetPos(llGetLocalPos() + delta);
if (touchPos != <0.0,0.0,0.0>) LastTouchPos = touchPos;
}
}
Pros: can click anywhere and the prim will follow. Can click and drag without any limit on speed.
Cons: only works with 1 prim at a time (in this script's current state), requires 2 prims, doesn't always drag directly under where your mouse is (again probably just the way the script is now, it needs some tweeks.) Put this script in the root and make that prim invisible, stretch it to however large of an area you want, and link it to another object you want to drag and make sure it's under the root object.
//written by Valent1ne
default
{
touch(integer total_number)
{
vector offset = <.58,.45,0>; //this sort of random vector is the offset, you'll have to play with it to get it to work just right. At least until I figure out a better way.
vector pos = llDetectedTouchUV(0) - offset;
llSetLinkPrimitiveParamsFast(LINK_ALL_CHILDREN, [PRIM_POSITION,pos]);
}
}
Tuesday, July 27, 2010
Remove inventory
Someone asked me tonight wanting to know how to get more than one script to delete, including itself. The answer was simple, have the other scripts delete first, then have the script self destruct. Remember LSL reads top to bottom, left to right. So if you have one function before the other, the one on the topmost line will run first.
llRemoveInventory("New Script 1");
llRemoveInventory("New Script 2");
llRemoveInventory(llGetScriptName());
Instructions
nucularsecrets@gmail.com
Any question is fair game, so don't be shy!
Featured
- OpenSim (1)
- Outline (1)
- Sculpties in Blender (1)
Followers
Contributors
Friends
Index
- PHP (1)
- changed (2)
- click and drag (1)
- dataserver (2)
- link_message (1)
- llAllowInventoryDrop (1)
- llAvatarOnSitTarget (1)
- llDetectedKey (2)
- llDetectedName (1)
- llDetectedTouchPos (1)
- llDetectedTouchUV (1)
- llGetOwner (2)
- llGetScriptName (1)
- llHTTPRequest (1)
- llListen (1)
- llParticleSystem (1)
- llRegionSay (1)
- llRemoveInventory (1)
- llRequestAgentData (2)
- llResetScript (1)
- llSay (3)
- llSetLinkPrimitiveParamsfast (2)
- llSetObjectName (1)
- llSetPos (1)
- llSetTimerEvent (1)
- llSitTarget (1)
- particles (1)
- timer (1)
- touch (1)
Blog Archive
-
►
2010
(18)
- ► Nov 21 - Nov 28 (1)
- ► Sep 19 - Sep 26 (1)
- ► Aug 15 - Aug 22 (1)
- ► Aug 8 - Aug 15 (1)
- ► Aug 1 - Aug 8 (1)
- ► Jul 25 - Aug 1 (1)
- ► Jul 4 - Jul 11 (1)
- ► Jun 13 - Jun 20 (1)
- ► May 23 - May 30 (3)
- ► May 16 - May 23 (5)
- ► May 2 - May 9 (2)
Copyright 2010 Nucular Secrets . Powered by Blogger
Blogger Templates created by Deluxe Templates Wordpress by thebookish